Upgrading to Laravel 8.x
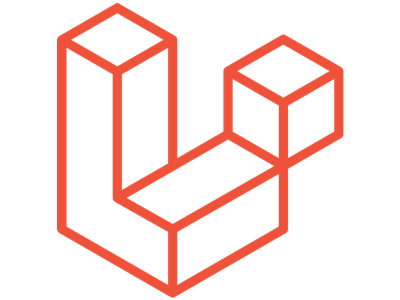
Laravel 8.x came out on 9/8/2020 and we wanted to create a post for how to update our applications to the newest version of Laravel.
A Disclaimer
Laravel 8 is brand new and because it has breaking changes it has the potential to break our applications so do not install this directly on a production application. We take no responsibility whatsoever for this post breaking anything.
As a general piece of advice, it’s a good idea to hold off a couple of weeks so bugs can get fixed before upgrading any production instances. But it’s okay to install the newest version in a development environment to try out new features and help find any bugs.
Upgrade Instructions
Laravel’s upgrade path is quite straightforward and all the steps can be found on Laravel’s website at https://laravel.com/docs/8.x/upgrade. It’s important to read the most current version of the upgrade documentation. It changed in the time between when we starting writing this post and when we published it.
As part of the upgrade to 8.x, several of Laravel’s first-party packages have been upgraded to support Laravel 8.x. If you’re using one of the packages listed below you should read the instructions on how to upgrade them (links are below) before finishing the upgrade to 8.x.
Create a New Branch
Any time we do a major upgrade, we have to assume it’s a test and we might encounter problems that we can’t get past. Because of this, we may have to abandon this process.
To do that easily, we can create a branch so that we can just delete the branch if everything doesn’t work out for us.
git checkout master
git checkout -b "laravel-8"
Change composer.json
The next thing we’re going to do is open our composer.json and make changes that need to be made to get to version 8.
We have to change.
- guzzlehttp/guzzle to ^7.0.1
- facade/ignition to ^2.3.6
- laravel/framework to ^8.0
- laravel/ui to ^3.0
- nunomaduro/collision to ^5.0
- phpunit/phpunit to ^9.0
We’ll end up with something like the following.
{
"name": "laravel/laravel",
"type": "project",
"description": "The Laravel Framework.",
"keywords": [
"framework",
"laravel"
],
"license": "MIT",
"require": {
"php": "^7.2.5",
"fideloper/proxy": "^4.2",
"fruitcake/laravel-cors": "^1.0",
"guzzlehttp/guzzle": "^7.0.1",
"laravel/framework": "^8.0",
"laravel/ui": "^3.0",
"laravel/tinker": "^2.0"
},
"require-dev": {
"facade/ignition": "^2.3.6",
"fzaninotto/faker": "^1.9.1",
"mockery/mockery": "^1.3.1",
"nunomaduro/collision": "^5.0",
"phpunit/phpunit": "^9.0"
},
Next, we’re going to run composer update
.
vagrant@ubuntu-xenial:/var/www$ composer update
Loading composer repositories with package information
Updating dependencies (including require-dev)
Package operations: 2 installs, 5 updates, 0 removals
- Installing psr/http-client (1.0.1): Loading from cache
- Updating guzzlehttp/guzzle (6.5.5 => 7.0.1): Loading from cache
- Installing graham-campbell/result-type (v1.0.1): Loading from cache
- Updating vlucas/phpdotenv (v4.1.8 => v5.1.0): Loading from cache
- Updating dragonmantank/cron-expression (v2.3.0 => 3.0.1): Loading from cache
- Updating laravel/framework (v7.28.0 => v8.0.0): Loading from cache
- Updating nunomaduro/collision (v4.2.0 => v5.0.2): Loading from cache
Package phpunit/php-token-stream is abandoned, you should avoid using it. No replacement was suggested.
Writing lock file
Generating optimized autoload files
> Illuminate\Foundation\ComposerScripts::postAutoloadDump
> @php artisan package:discover --ansi
Discovered Package: facade/ignition
Discovered Package: fideloper/proxy
Discovered Package: fruitcake/laravel-cors
Discovered Package: laravel/tinker
Discovered Package: nesbot/carbon
Discovered Package: nunomaduro/collision
Package manifest generated successfully.
Now that everything has been downloaded successfully we want to make sure we commit composer.json and composer.lock to our repository. composer.lock is important because it’s the file that composer generates to make sure that we’re always installing the same version of the packages when we run composer install
.
Now that we have upgraded to Laravel 8 we need to run all of our tests and check critical pages and make sure that nothing broke. Once we feel confident that it’s working we can merge the branch into master
git checkout master
git merge "laravel-8"
Next Steps
There are a couple of things we need to focus on due to this upgrade that have been marked as high impact changes in the upgrade documentation.
Model Factories
The first is that the model factories are now classes so we’ll have to go through and move them to the new structure.
There is a library that allows us to continue to use the old factories but we should bite the bullet and upgrade them as soon as possible.
Name Changes
The first is that the retryAfter
method for any queued jobs, mailers, notifications, and listeners has been renamed to backoff
. There isn’t an automated way to fix these and we’re going to have to manually update them. This can be done using a global search and replace.
The timeoutAt
property for queued jobs, notifications, and listeners has been renamed to retryUntil
. It’s again that’s one of these things we should fix using a global search and replace.
Paginator
The last change is that the paginator is now using the Tailwind CSS framework instead of Bootstrap. We have to make a change to our AppServicesProvider.php
file to force it to use the Bootstrap layout.
use Illuminate\Pagination\Paginator;
Paginator::useBootstrap();
Enjoy The Upgrade
Hopefully, the upgrade has gone smoothly and our application is now up to date with Laravel 8.x.
Scott Keck-Warren
Scott is the Director of Technology at WeCare Connect where he strives to provide solutions for his customers needs. He's the father of two and can be found most weekends working on projects around the house with his loving partner.
Top Posts
- Working With Soft Deletes in Laravel (By Example)
- Fixing CMake was unable to find a build program corresponding to "Unix Makefiles"
- Upgrading to Laravel 8.x
- Get The Count of the Number of Users in an AD Group
- Multiple Vagrant VMs in One Vagrantfile
- Fixing the "this is larger than GitHub's recommended maximum file size of 50.00 MB" error
- Changing the Directory Vagrant Stores the VMs In
- Accepting Android SDK Licenses From The OSX Command Line
- Fixing the 'Target class [config] does not exist' Error
- Using Rectangle to Manage MacOS Windows