Truthy and Falsy in PHP
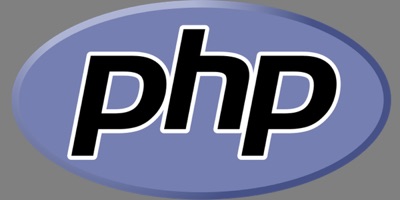
In PHP it’s fairly common to see the if
statement.
$booleanValue = true;
if ($booleanValue) {
doSomething();
}
if (!$booleanValue) {
dontDoSomethingElse();
}
We run across these all the time and can easily understand what happens when we pass them a true
or false
value. However what happens when the condition of the if
statement is an object, a string, or a number?
What Is Truthy/Falsy?
Loosely typed languages like PHP allow us to use any kind of variable as if it was a boolean value in our if
statements.
$valueString = 'hello';
if ($valueString) {
doSomething();
}
When PHP needs to evaluate the if
statement it converts our variable into something is can represent as a boolean. Ideally this would be a true
or false
but in PHP it will convert any variable into something it can represent as one of those two values. This is where “truthy” and “falsy” come in. A variable that isn’t true
or false
can be truthy or falsy.
Some Examples
Luckily for us there’s a logical consistency with how PHP type juggles our variables into truthy and falsy. For the most part the logic is that anything “empty” is falsy and anything with a value is truthy.
Numbers
0
is falsy but 1
or -1
are truthy.
<?php
if (0) {
// not here
} else {
echo "False";
}
if (1) {
echo "True";
}
if (-1) {
echo "True";
}
Arrays
Empty arrays are falsy but arrays with a value are truthy.
<?php
if ([]) {
// not here
} else {
echo "False";
}
if (['a value']) {
echo "True";
}
Strings
Empty strings are falsy but strings with a value are truthy.
<?php
if ('') {
// not here
} else {
echo "False";
}
if ('a value') {
echo "True";
}
Null
null
values are falsy.
<?php
if (null) {
// not here
} else {
echo "False";
}
Scott Keck-Warren
Scott is the Director of Technology at WeCare Connect where he strives to provide solutions for his customers needs. He's the father of two and can be found most weekends working on projects around the house with his loving partner.
Top Posts
- Working With Soft Deletes in Laravel (By Example)
- Fixing CMake was unable to find a build program corresponding to "Unix Makefiles"
- Upgrading to Laravel 8.x
- Get The Count of the Number of Users in an AD Group
- Multiple Vagrant VMs in One Vagrantfile
- Fixing the "this is larger than GitHub's recommended maximum file size of 50.00 MB" error
- Changing the Directory Vagrant Stores the VMs In
- Accepting Android SDK Licenses From The OSX Command Line
- Fixing the 'Target class [config] does not exist' Error
- Using Rectangle to Manage MacOS Windows