PHP_CodeSniffer
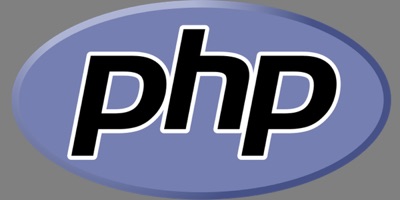
In our previous article, we discussed why we need a coding standard and why we should be using the PSR-2 or PSR-12 coding standards. In this article, we’re going to discuss a tool we can use to verify that our code is using that standard and correct it if it’s not.
Using PHP_CodeSniffer to Check and Correct Your Code
The tool we’re going to use for this process is the PHP_CodeSniffer Project. The PHP_CodeSniffer project comprises two different scripts. The PHP Code Sniffer (phpcs
) script is used to detect violations in our code and the PHP Code Beautifier and Fixer (phpcbf
) is used to automatically fix the violation (at least most of them).
To find violations in our code these tools use classes written in PHP called sniffs. That’s why it’s called PHP_CodeSniffer.
Most people don’t need to know this but in future articles, we’ll reference sniffs so you can say we didn’t tell you.
Per the PHP_CodeSniffer instructions, the easiest way to install these tools is to download them directly to our computer using something like curl
or wget
.
The passage below is taken from their GitHub repository.
# Download using curl
curl -OL https://squizlabs.github.io/PHP_CodeSniffer/phpcs.phar
curl -OL https://squizlabs.github.io/PHP_CodeSniffer/phpcbf.phar
# Or download using wget
wget https://squizlabs.github.io/PHP_CodeSniffer/phpcs.phar
wget https://squizlabs.github.io/PHP_CodeSniffer/phpcbf.phar
# Then test the downloaded PHARs
php phpcs.phar -h
php phpcbf.phar -h
We prefer using the composer package to install it into just our project because it will then be installed for anyone working on our project and at the same version.
composer require --dev "squizlabs/php_codesniffer=*"
Let’s look at an example. This is our User
class and it has a couple of places where the formatting doesn’t align with the PSR12 standard.
<?php
namespace App;
class a_User{
public function aFunctionWithFormattingIssue(){
echo true?"show":"don't";
}
}
To see where our problems are we’re going to run the phpcs
script against it.
$ ./vendor/bin/phpcs --standard=PSR12 app/User.php
FILE: /var/www/app/User.php
-----------------------------------------------------------------------------------
FOUND 10 ERRORS AFFECTING 5 LINES
-----------------------------------------------------------------------------------
1 | ERROR | [x] Header blocks must be separated by a single blank line
2 | ERROR | [x] Header blocks must be separated by a single blank line
3 | ERROR | [ ] Class name "a_User" is not in PascalCase format
3 | ERROR | [x] Opening brace of a class must be on the line after the definition
4 | ERROR | [x] Opening brace should be on a new line
5 | ERROR | [x] Line indented incorrectly; expected at least 8 spaces, found 5
5 | ERROR | [x] Expected at least 1 space before "?"; 0 found
5 | ERROR | [x] Expected at least 1 space after "?"; 0 found
5 | ERROR | [x] Expected at least 1 space before ":"; 0 found
5 | ERROR | [x] Expected at least 1 space after ":"; 0 found
-----------------------------------------------------------------------------------
PHPCBF CAN FIX THE 9 MARKED SNIFF VIOLATIONS AUTOMATICALLY
-----------------------------------------------------------------------------------
Time: 174ms; Memory: 8MB
Yikes, that’s a lot of issues we need to fix. Thankfully, the phpcbf
can come to our rescue and fix most of them for us.
$ ./vendor/bin/phpcbf --standard=PSR12 app/User.php
PHPCBF RESULT SUMMARY
----------------------------------------------------------------------
FILE FIXED REMAINING
----------------------------------------------------------------------
/var/www/app/User.php 9 1
----------------------------------------------------------------------
A TOTAL OF 9 ERRORS WERE FIXED IN 1 FILE
----------------------------------------------------------------------
Time: 344ms; Memory: 8MB
Notice how it says it fixed nine errors but there’s one remaining? We’ll come back to that in a minute.
Let’s see what our User
file looks like now that it’s been corrected.
<?php
namespace App;
class a_User
{
public function aFunctionWithFormattingIssue()
{
echo true ? "show" : "don't";
}
}
Mostly what we fixed were spacing issues so it’s no surprise that phpcbf
could do it automatically.
Let’s see what our final error is.
$ ./vendor/bin/phpcs --standard=PSR12 app/User.php
FILE: /var/www/app/User.php
----------------------------------------------------------------------
FOUND 1 ERROR AFFECTING 1 LINE
----------------------------------------------------------------------
5 | ERROR | Class name "a_User" is not in PascalCase format
----------------------------------------------------------------------
Time: 169ms; Memory: 8MB
In this case, the name of our class should be in PascalCase but it’s not. This is an example of a rule that we have to manually fix. Once we do this phpcs
won’t report any problems:
$ ./vendor/bin/phpcs --standard=PSR12 app/User.php
$
Using an Editor Plugin
We never want to do anything manually that can be done automatically. To that end, the best place to run static code analysis tools is as close to when we make our change as possible. In this case, we absolutely should use whatever methods our editor supports to run phpcs
and phpcbf
as soon as we’re written our code.
Ideally, we would have our editor display the errors as we are writing the code as well as have it set up to automatically reformat the code so we don’t need to do anything manually.
What You Need To Know
- The PHP_CodeSniffer Project provides the
phpcs
andphpcbf
scripts phpcs
shows us our errorsphpcbf
fixes them automatically
Scott Keck-Warren
Scott is the Director of Technology at WeCare Connect where he strives to provide solutions for his customers needs. He's the father of two and can be found most weekends working on projects around the house with his loving partner.
Top Posts
- Working With Soft Deletes in Laravel (By Example)
- Fixing CMake was unable to find a build program corresponding to "Unix Makefiles"
- Upgrading to Laravel 8.x
- Get The Count of the Number of Users in an AD Group
- Multiple Vagrant VMs in One Vagrantfile
- Fixing the "this is larger than GitHub's recommended maximum file size of 50.00 MB" error
- Changing the Directory Vagrant Stores the VMs In
- Accepting Android SDK Licenses From The OSX Command Line
- Fixing the 'Target class [config] does not exist' Error
- Using Rectangle to Manage MacOS Windows