Working With Cookies in PHP
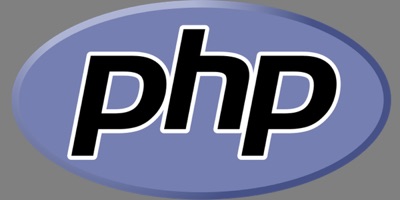
A core functionality of any modern web application is keeping track of our users between web requests. We could add a unique identifier to every URL but this makes our URLs hard to generate, hard to read, and hard to share. Thankfully, every modern browser supports cookies to keep track of our users between requests.
In this article, we’ll discuss how to use cookies in our PHP applications.
What is a Cookie?
A cookie is a small file (maximum of 4KB) that allows us as developers to store information on our user’s computer. This information will then be sent back to our web server whenever the user makes a request. A cookie is generally used to keep track of information such as a unique identifier of the person requesting the resource so we can build the page specifically tailored to them.
Cookies have a bad rap because they’re used to track users across multiple sites for advertisers which is why every site on the internet needs to have a banner telling its users that it uses cookies. Those types of cookies are referred to as 3rd-party cookies because they’re being requested from a 3rd-party server, not the user or web server directly involved in the request.
We should not store sensitive data in cookies since it could be potentially manipulated by a malicious user. To store sensitive data securely we should use sessions instead. We’ll discuss sessions in our next article.
Cookies are transmitted back to the server in the HTTP headers. Using HTTP will encrypt the cookie data but HTTP won’t.
Cookies in PHP
PHP has built-in support for cookies all the way back to version 4.0. It does this with several helper functions and a global variable.
We must set our cookies before we start outputting the HTML of our page. This is because cookies are set in the header section of the HTTP request. PHP’s header()
function has the same limitation.
Writing Cookie Data
PHP’s cookie support is essentially a key-value store. We use the setcookie()
function to set data.
setcookie(
string $name,
string $value = "",
int $expires_or_options = 0,
string $path = "",
string $domain = "",
bool $secure = false,
bool $httponly = false
): bool
There are an annoyingly large number of optional parameters to the setcookie()
function. All of the options except for the name
are optional.
The most important parameters are the name, which is what we’re calling the cookie, and the value, which is what the value is.
$value = 'our value';
setcookie("OurCookie", $value);
We can also set the expires parameter which allows us to say how long the client computer should keep the cookie. 0 is the default which means it’s valid until the browser is closed. Otherwise, we’ll send a UNIX timestamp for when the cookie expires. For example, we might want to keep the cookie for 30 days.
setcookie("OurCookie", $value, time() + 60 * 60 * 24 * 30);
The path parameter specifies where the cookie is valid on the domain. As an example of that, we might have one domain supporting multiple applications. There might be a finance application in the “/finance” directory and the sales application in the “/sales” directory.
We can use this parameter to allow us to say this cookie is only valid in the “/finance” directory or only valid in the “/sales” directory.
setcookie("OurCookie", $value, time() + 3600, "/finance/");
setcookie("OurCookie", $value, time() + 3600, "/sales/");
The next parameter is the domain, which allows us to set the domain for which the cookie is valid. We can set it to test.com which will allow for test.com and all of its subdomains. We can set it to finance.test.com and so it will work for finance.test.com but it won’t work for test.com and it won’t work for sales.test.com.
The last two parameters are the secure and httponly only parameters, these allow us to determine whether the cookie should be sent only over HTTPS or only over HTTP connections. By default, it’s sent over both.
Reading Cookie Data
To read cookie data we use the $_COOKIE
global variable. It’s an associative array where the key is the name that we used in setcookie()
and because it’s already an array we can use the PHP array functions that we already know.
For example the isset()
function
if(!isset($_COOKIE["OurCookie"])) {
echo "Cookie named 'OurCookie' is not set!";
} else {
echo "Cookie 'OurCookie' is set!<br>";
echo "Value is: " . $_COOKIE["OurCookie"];
}
To modify a cookie we call setcookie()
again on the same name.
$value = 'our new value';
setcookie("OurCookie", $value);
Deleting Cookie Values
To delete a cookie, use the setcookie() function with an expiration date in the past:
setcookie("OurCookie", $value, time() - 60);
The Downsides
There are some downsides to cookies. The first is that they’re not always secure and they can be tampered with. A user could put data in there that we don’t want and they could send it to our server. We’re limited to 4 KB which might seem like a lot but it does have its limitations and we have to transmit the whole cookie with every request which can add up.
What You Need To Know
- Cookies allow us to store information on user’s computer
- Less than 4KB
- PHP 4.0 and greater have supported
- setcookie($name, $value)
- $_COOKIE global varibles
Scott Keck-Warren
Scott is the Director of Technology at WeCare Connect where he strives to provide solutions for his customers needs. He's the father of two and can be found most weekends working on projects around the house with his loving partner.
Top Posts
- Working With Soft Deletes in Laravel (By Example)
- Fixing CMake was unable to find a build program corresponding to "Unix Makefiles"
- Upgrading to Laravel 8.x
- Get The Count of the Number of Users in an AD Group
- Multiple Vagrant VMs in One Vagrantfile
- Fixing the "this is larger than GitHub's recommended maximum file size of 50.00 MB" error
- Changing the Directory Vagrant Stores the VMs In
- Accepting Android SDK Licenses From The OSX Command Line
- Fixing the 'Target class [config] does not exist' Error
- Using Rectangle to Manage MacOS Windows