The PSR Coding Standards
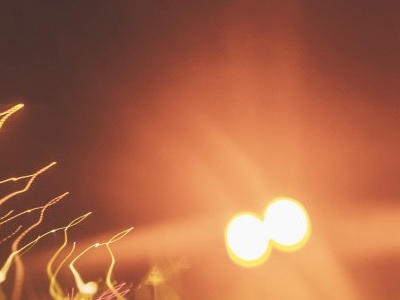
There are as many ways of formatting our code as there are developers who write it. When we’re working on a team there needs to be a common way to format the code to maximize team cohesion.
By having a coding standard we can define exactly how we expect the code to be formatted and make it easier for everyone to read. In this article we’ll discuss why we need a coding standard, what should be included, and why we should start by looking at the PSR family of standards.
What Are Coding Standards?
Coding standards are a set of coding rules, conventions, and best practices that we should adhere to as we’re writing our source code. They should help us write cleaner, more understandable code.
Why Have A Coding Standard?
As developers, we’ll use these coding standards to inform how we write our code every day. Instead of each developer contributing code using their own set of preferences, they will use the agreed-upon coding standard.
The biggest gain here is that this makes sure that the code is in a consistent style and that parts of the code written by different developers don’t look different. Not only does it make the code easier for every developer to understand, but as each developer looks at the code they’ll know what to expect.
Things that should be included in a coding standard include but are not limited to:
- Where brackets are placed
- If tabs or spaces are used in indentation
- How variables should be formatted (camelCase vs snake_case vs PascalCase)
- Spacing (
$check ? “Yes” : “No”
vsecho $check?“Yes”:“No”
) - How to name classes and functions
Some of these items can be validated using automated tools and some of them need to be done manually through code reviews. In our next article in this series, we will discuss how to use an automated tool to check our code against a standard.
How to Name Things?
Naming things is a challenge. As developers, we need to make sure that we’re doing our best to name things in a way that others will be able to understand our intent.
We always wanna make sure that as we’re naming things someone can understand what is going on now, a week from now, and a year from now. Sometimes, it’s someone else reading the code but a lot of the time it will be ourselves. We want to make sure that we give ourselves the best possible setup to make any changes and debug problems.
As a team, it’s always a good idea to have a discussion and decide on standards for how to name functions, classes, and variables. This will increase the chances that everyone’s code will be consistent.
How To Pick a Standard
Just as there are a wide variety of developer preferences in coding there are also a wide variety of options for coding standards.
When picking a coding standard we have three options. The first is that we can create a coding standard from scratch. The second is that we can borrow a coding standard that already exists and use it as is. Finally, we can borrow a coding standard that exists and then add or remove rules that don’t fit our team’s environment.
Over the years we’ve used all three approaches and we found the best approach is it start with an existing coding standard and then modify it for our team. This allows us to not get stuck making small decisions like bracket placement and instead focus on the items that can help our team be as efficient as possible.
There are lots of different coding standards that we can pick from including:
Let’s talk about the one we prefer to use.
The PSR Family of Standards
The PSR family of standards was created by the PHP Framework Interop Group (PHP-FIG). The benefit of using this family of standards is that if our application is using a framework that framework will most likely be using the same standard. It can be problematic if the framework we’re using doesn’t match the standard we’ve picked.
The PSR family of standards comprises three different documents that define how our code should be developed.
PSR-1: Basic Coding Standards
The PSR-1 coding standard was the original coding standard set forth by the PHP-FIG. It has basic rules like how files should be formatted.
This is no longer a standard we should use directly. Instead, we should use the PSR-2 or PSR-12 standards because they use PSR-1 as a starting point and then add more to it.
For a full listing of the rules see: https://www.php-fig.org/psr/psr-1/
PSR-2: Coding Style Guide
The PSR-2 standard was the first attempt at reducing the cognitive friction when scanning code from different authors. It does so by listing out a wide variety of rules and expectations for how to format our PHP code.
Examples of this include using four spaces for indenting and how a line should be 80 characters or less but never more than 120 characters.
As we said before this extends the PSR-1 standard.
The PSR-2 standard has been replaced by the PSR-12 standard so for an application supporting the currently supported versions of the PHP language, it’s best to use the PSR-12 standards.
For a full listing of the rules see: https://www.php-fig.org/psr/psr-2/
PSR-12: Extended Coding Style
The PSR-12 standard was the second attempt at reducing the cognitive friction when scanning code from different authors. This is the second attempt because the PSR-2 was accepted in 2012 and needed to be updated for the changes in the PHP core language.
The PSR-12 standard also extends the PSR-1 standard.
Our preference is to use the PSR-12 standard as it does have those additional items.
For a full listing of the rules see: https://www.php-fig.org/psr/psr-12/
Legacy Code
When we’re adding a coding standard to an existing project there are essentially two ways we can do this.
The first is that we can run a pass over the code to rewrite everything all at once. This is an excellent way to get everything up-to-date quickly but this can be a challenge to implement when multiple people are working in the code base and there are multiple open PRs/branches that need to be maintained (trust us the conflicts this creates can be overwhelming).
The second way is to apply the new standard to new files and then clean up all the files as they are worked on.
Our decision here will depend on several factors including how many open PRs the project has and how much churn there is in the codebase. Our preference is to only apply the new standard to new files and then clean up code slowly. We’ve even made it a daily task for a developer to clean up one percent of the files a day. This reduces the chance of us having conflicts but still moves us forward.
What You Need To Know
- Coding standards are a set of coding rules, conventions, and best practices
- Help make code easier to read and thus easier to maintain
- The PHP-FIG has a series of standards that are a great starting point
Scott Keck-Warren
Scott is the Director of Technology at WeCare Connect where he strives to provide solutions for his customers needs. He's the father of two and can be found most weekends working on projects around the house with his loving partner.
Top Posts
- Working With Soft Deletes in Laravel (By Example)
- Fixing CMake was unable to find a build program corresponding to "Unix Makefiles"
- Upgrading to Laravel 8.x
- Get The Count of the Number of Users in an AD Group
- Multiple Vagrant VMs in One Vagrantfile
- Fixing the "this is larger than GitHub's recommended maximum file size of 50.00 MB" error
- Changing the Directory Vagrant Stores the VMs In
- Accepting Android SDK Licenses From The OSX Command Line
- Fixing the 'Target class [config] does not exist' Error
- Using Rectangle to Manage MacOS Windows