What the F*ck Is With All the Artisan Commands: Route Commands
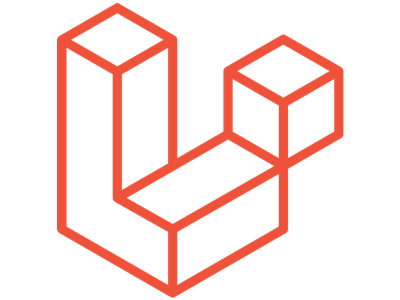
There are three route commands that we need to quickly cover in order to be completionist in this process of going over every artisan command.
route:list
Why It Exists
route:list
allows you to view a listing of all the routes that have been defined in your application.
ubuntu@ubuntu-xenial:/var/www$ php artisan route:list
+--------+-----------+-------------------------+------------------+------------------------------------------------+------------- +
| Domain | Method | URI | Name | Action | Middleware |
+--------+-----------+-------------------------+------------------+------------------------------------------------+------------- +
| | GET|HEAD | / | | Closure | web |
| | GET|HEAD | api/user | | Closure | api,auth:api |
| | GET|HEAD | home | home | App\Http\Controllers\HomeController@index | web,auth |
| | GET|HEAD | projects | projects.index | App\Http\Controllers\ProjectController@index | web,auth |
| | POST | projects | projects.store | App\Http\Controllers\ProjectController@store | web,auth |
| | GET|HEAD | projects/create | projects.create | App\Http\Controllers\ProjectController@create | web,auth |
| | GET|HEAD | projects/{project} | projects.show | App\Http\Controllers\ProjectController@show | web,auth |
| | PUT|PATCH | projects/{project} | projects.update | App\Http\Controllers\ProjectController@update | web,auth |
| | DELETE | projects/{project} | projects.destroy | App\Http\Controllers\ProjectController@destroy | web,auth |
| | GET|HEAD | projects/{project}/edit | projects.edit | App\Http\Controllers\ProjectController@edit | web,auth |
+--------+-----------+-------------------------+------------------+------------------------------------------------+------------- +
When Should You Use It
route:list
is helpful for debugging when a route isn’t working and for seeing what’s available.
--compact
The defaults on the route:list
command is extremely verbose. It’s so verbose that it doesn’t even fit into this post without you scrolling over to see it’s full output (sorry people on mobile). There is a --compact
parameter that reduces the amount of information that’s displayed. You can also do this with the --columns
parameter but this is a quick way to see some of the important pieces.
vagrant@ubuntu-xenial:/var/www$ php artisan route:list --compact
+-----------+-------------------------+------------------------------------------------+
| Method | URI | Action |
+-----------+-------------------------+------------------------------------------------+
| GET|HEAD | / | Closure |
| GET|HEAD | api/user | Closure |
| GET|HEAD | home | App\Http\Controllers\HomeController@index |
| GET|HEAD | projects | App\Http\Controllers\ProjectController@index |
| POST | projects | App\Http\Controllers\ProjectController@store |
| GET|HEAD | projects/create | App\Http\Controllers\ProjectController@create |
| DELETE | projects/{project} | App\Http\Controllers\ProjectController@destroy |
| PUT|PATCH | projects/{project} | App\Http\Controllers\ProjectController@update |
| GET|HEAD | projects/{project} | App\Http\Controllers\ProjectController@show |
| GET|HEAD | projects/{project}/edit | App\Http\Controllers\ProjectController@edit |
+-----------+-------------------------+------------------------------------------------+
--method
, --name
, and --path
The route:list
command also has --method
, --name
, and --path
arguments which allow you to filter the output of the command on the method, name, and path of the route so it’s easier to digest.
vagrant@ubuntu-xenial:/var/www$ php artisan route:list --compact --path=project
+-----------+-------------------------+------------------------------------------------+
| Method | URI | Action |
+-----------+-------------------------+------------------------------------------------+
| GET|HEAD | projects | App\Http\Controllers\ProjectController@index |
| POST | projects | App\Http\Controllers\ProjectController@store |
| GET|HEAD | projects/create | App\Http\Controllers\ProjectController@create |
| GET|HEAD | projects/{project} | App\Http\Controllers\ProjectController@show |
| PUT|PATCH | projects/{project} | App\Http\Controllers\ProjectController@update |
| DELETE | projects/{project} | App\Http\Controllers\ProjectController@destroy |
| GET|HEAD | projects/{project}/edit | App\Http\Controllers\ProjectController@edit |
+-----------+-------------------------+------------------------------------------------+
The Route Cache
Laravel provides a couple caches but it provides two commands to cache your routes in order to improve performance.
route:cache
The route:cache
command allows you to clear and create the route cache.
php artisan route:cache
Route cache cleared!
Routes cached successfully!
route:clear
The route:clear
command allows you to just clear the route cache. This is a helpful command for when your routes aren’t working correctly and you need to troubleshoot them.
php artisan route:clear
Route cache cleared!
Hopefully this has been as helpful to you as it has to me. Check back soon for more artisan commands.
Scott Keck-Warren
Scott is the Director of Technology at WeCare Connect where he strives to provide solutions for his customers needs. He's the father of two and can be found most weekends working on projects around the house with his loving partner.
Top Posts
- Working With Soft Deletes in Laravel (By Example)
- Fixing CMake was unable to find a build program corresponding to "Unix Makefiles"
- Upgrading to Laravel 8.x
- Get The Count of the Number of Users in an AD Group
- Multiple Vagrant VMs in One Vagrantfile
- Fixing the "this is larger than GitHub's recommended maximum file size of 50.00 MB" error
- Changing the Directory Vagrant Stores the VMs In
- Accepting Android SDK Licenses From The OSX Command Line
- Fixing the 'Target class [config] does not exist' Error
- Using Rectangle to Manage MacOS Windows