Laravel
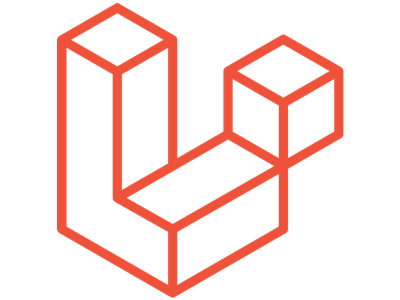
Ready to level up your knowledge of Laravel? Checkout out these articles!
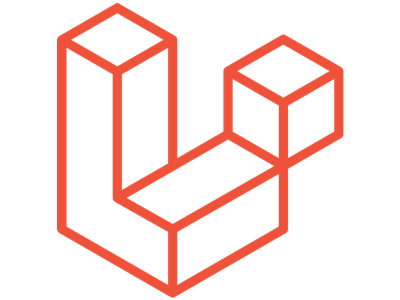
How To Work With Enumerations in Laravel Framework 9
Laravel 9 was released on February 8th, 2022 and it came with some nice new features that we want to highlight.
In this article, we’ll discuss how to work with enumerations in Laravel Framework 9+
View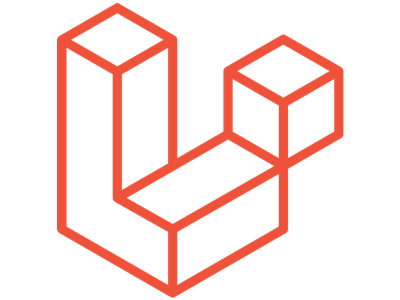
Upgrading From Laravel 8.x to Laravel 9.x
Laravel 9 was released on 2/8/2022 and in this article, we’ll describe how to update our applications from Laravel 8 to Laravel 9.
If you’re upgrading from a previous version make sure you check out our article on how to upgrade to Laravel 8. You’ll have to upgrade through each version one at a time.
View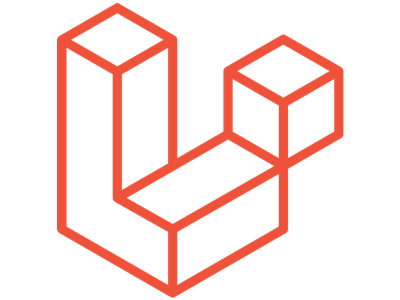
Working With Soft Deletes in Laravel 8 and 9 (By Example)
The annoying thing about deleting data from a database is that it’s gone forever. We can’t even look at the data to see if we needed it because it’s gone. If we need the data back, our only solution will be to restore a backup and hope we have the backup just before it was deleted so the loss is minimized.
Thankfully, Laravel provides a built-in feature that allows us to flag database rows as deleted without actually deleting them from the database. This article/video discusses how to use Soft Deletes in Laravel.
View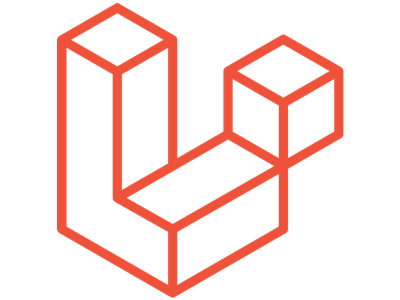
What the F*ck Is With All the Artisan Commands: Schedules
Scheduled tasks will be a core part of our application’s life cycle. We need to be able to send invoices every month and reminder users of upcoming items. Laravel provides a clean interface for quickly setting up scheduled tasks.
View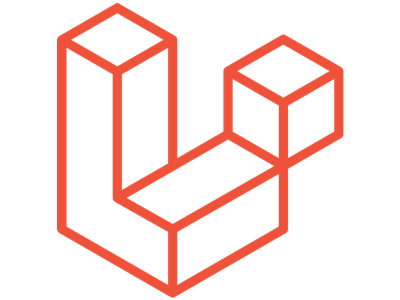
Running Unit Tests With Artisan's Test Runner
In the 7.x branch, Laravel added an artisan test
command which acts as a wrapper for PHPUnit. It provides information about the tests as they’re running and stops on the first failure unlike PHPUnit’s default behavior of running all the tests and showing all the failures. It also provides a nice output when the tests fail.
The following article will provide a brief overview of how we can use it and quickly compare it to phpunit
.
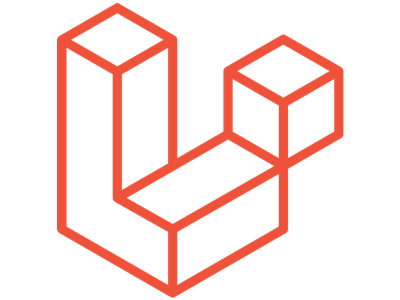
Creating Reusable Bootstrap Elements With Laravel's Make:Component
Bootstrap is a good system for getting an application’s layout started and it comes with lots of helpful user interface elements that we can use in our application. The downside, is there tends to be a lot of duplication in our source code to support these elements. Thankfully, Laravel added components in the 7.x branch which will allow us to reduce that duplication.
View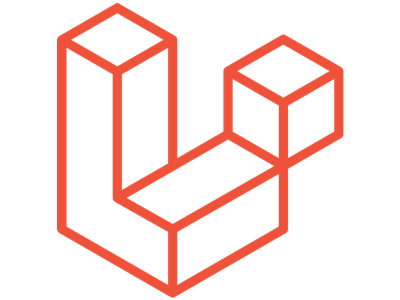
Fixing the 'Target class [config] does not exist' Error
The other day I pushed a new test to our Jenkins server and I received the following error.
1) Tests\Unit\NewTest::testIfDeletedBeforeStartDateThenWrongHire
Illuminate\Contracts\Container\BindingResolutionException: Target class [config] does not exist.
/var/www/vendor/laravel/framework/src/Illuminate/Container/Container.php:805
/var/www/vendor/laravel/framework/src/Illuminate/Container/Container.php:681
/var/www/vendor/laravel/framework/src/Illuminate/Container/Container.php:629
/var/www/vendor/laravel/framework/src/Illuminate/Foundation/Application.php:769
/var/www/vendor/laravel/framework/src/Illuminate/Container/Container.php:1245
/var/www/vendor/laravel/framework/src/Illuminate/Database/DatabaseManager.php:270
/var/www/vendor/laravel/framework/src/Illuminate/Database/DatabaseManager.php:101
/var/www/vendor/laravel/framework/src/Illuminate/Database/DatabaseManager.php:77
/var/www/vendor/laravel/framework/src/Illuminate/Database/Eloquent/Model.php:1253
/var/www/vendor/laravel/framework/src/Illuminate/Database/Eloquent/Model.php:1219
/var/www/vendor/laravel/framework/src/Illuminate/Database/Eloquent/Model.php:1051
/var/www/vendor/laravel/framework/src/Illuminate/Database/Eloquent/Model.php:968
/var/www/vendor/laravel/framework/src/Illuminate/Database/Eloquent/Model.php:1004
/var/www/vendor/laravel/framework/src/Illuminate/Database/Eloquent/Model.php:957
/var/www/vendor/laravel/framework/src/Illuminate/Database/Eloquent/Model.php:1618
/var/www/vendor/laravel/framework/src/Illuminate/Database/Eloquent/Model.php:1630
/var/www/tests/TestCase.php:152
/var/www/tests/TestCase.php:116
/var/www/tests/TestCase.php:70
/var/www/tests/Unit/NewTest.php:17
/home/jenkins/.composer/vendor/phpunit/phpunit/src/TextUI/Command.php:200
/home/jenkins/.composer/vendor/phpunit/phpunit/src/TextUI/Command.php:159
Caused by
ReflectionException: Class config does not exist
/var/www/vendor/laravel/framework/src/Illuminate/Container/Container.php:803
/var/www/vendor/laravel/framework/src/Illuminate/Container/Container.php:681
/var/www/vendor/laravel/framework/src/Illuminate/Container/Container.php:629
/var/www/vendor/laravel/framework/src/Illuminate/Foundation/Application.php:769
/var/www/vendor/laravel/framework/src/Illuminate/Container/Container.php:1245
/var/www/vendor/laravel/framework/src/Illuminate/Database/DatabaseManager.php:270
/var/www/vendor/laravel/framework/src/Illuminate/Database/DatabaseManager.php:101
/var/www/vendor/laravel/framework/src/Illuminate/Database/DatabaseManager.php:77
/var/www/vendor/laravel/framework/src/Illuminate/Database/Eloquent/Model.php:1253
/var/www/vendor/laravel/framework/src/Illuminate/Database/Eloquent/Model.php:1219
/var/www/vendor/laravel/framework/src/Illuminate/Database/Eloquent/Model.php:1051
/var/www/vendor/laravel/framework/src/Illuminate/Database/Eloquent/Model.php:968
/var/www/vendor/laravel/framework/src/Illuminate/Database/Eloquent/Model.php:1004
/var/www/vendor/laravel/framework/src/Illuminate/Database/Eloquent/Model.php:957
/var/www/vendor/laravel/framework/src/Illuminate/Database/Eloquent/Model.php:1618
/var/www/vendor/laravel/framework/src/Illuminate/Database/Eloquent/Model.php:1630
/var/www/tests/TestCase.php:152
/var/www/tests/TestCase.php:116
/var/www/tests/TestCase.php:70
/var/www/tests/Unit/NewTest.php:17
/home/jenkins/.composer/vendor/phpunit/phpunit/src/TextUI/Command.php:200
/home/jenkins/.composer/vendor/phpunit/phpunit/src/TextUI/Command.php:159
The issue was that I had forgotten to include parent::setup()
in the test class’ setup()
function.
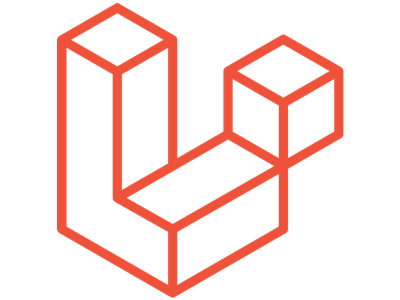
What the F*ck Is With All the Artisan Commands: Queues
Work queues allow you to perform tasks that are slow or error-prone outside of the current user’s request to improve their experience with your site. Read on to learn how you can use work queues in Laravel for your projects.
View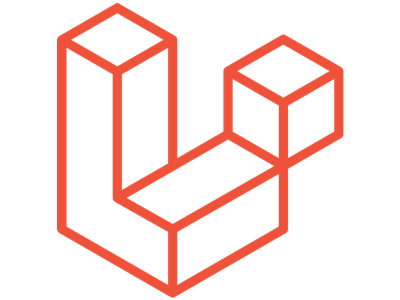
What the F*ck Is With All the Artisan Commands: Route Commands
There are three route commands that we need to quickly cover in order to be completionist in this process of going over every artisan command.
View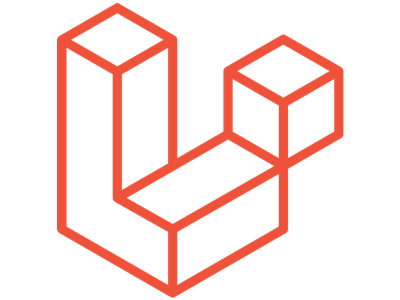
What the F*ck Is With All the Artisan Commands: Seeders
Now that we have discussed creating factories, we can look at how to use these factories to seed our database with test data.
View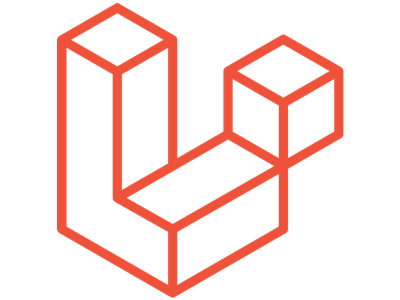
What the F*ck Is With All the Artisan Commands: Factories
Now that we know how to create tests, it’s time we looked at how we can use factories to generate test data quickly and easily.
ViewConverting Laravel 6.x Migrations to MySQL
I’ve had trouble knowing what to put in my migrations’ up()
and down()
functions. The Laravel Docs include a lot of information but I wanted a quick reference guide for myself to convert Laravel migrations to MySQL commands because that’s what I’m used to. :-)
I also spend some time compiling this into a Cheetsheet if you want a printable version of this post.
ViewDebugging the Eloquent Query Builder
The other day I had an Eloquent query that wasn’t returning what I expected it to. I started looking for a way to determine what was happening “under the hood” so to speak. It turns out it’s very simple.
ViewDefining Relationships In Laravel 5.6 and 6.x
I wanted to take a quick break in my barrage of Artisan posts to write about defining one-to-many and many-to-many relationships in Laravel’s Eloquent. Eloquent also supports one-to-one relationships but I felt like those are used so rarely that I could skip it.
ViewWhat the F*ck Is With All the Artisan Commands: Development Helpers
Here are two useful commands that you can use every day and one that’s good for getting yourself setup.
ViewWhat the F*ck Is With All the Artisan Commands: Quickies
As I’ve been organizing the flow of this series there’s a random set of commands that don’t really have a good home. I thought I would cover them now so they’re out of the way.
ViewWhat the F*ck Is With All the Artisan Commands: migrate
Now that we’ve learned how to create our migrations it’s time to discuss how to work with them using the migrate
family of commands.
What the F*ck Is With All the Artisan Commands: make:migration
Let’s start with my favorite feature so far: database migrations. I’m going to start with this command because I think it’s one of the more critical components and because the next article will involve working with them. :-)
ViewWhat the F*ck Is With All the Artisan Commands
At work, we’re moving our application from Zend Framework to Laravel. I don’t have much experience with Laravel so I’ve been working my way through Laravel 5.7 From Scratch . I’ve been amazed at how easy it is to work with Laravel but I’m overwhelmed by Artisan’s list of available commands. My goal over the next several weeks is to look at each command and determine why it’s there and when the command should be used. Mostly due to my own self interested but hopefully others will find it helpful.
ViewScott Keck-Warren
Scott is the Director of Technology at WeCare Connect where he strives to provide solutions for his customers needs. He's the father of two and can be found most weekends working on projects around the house with his loving partner.
Top Posts
- Working With Soft Deletes in Laravel (By Example)
- Fixing CMake was unable to find a build program corresponding to "Unix Makefiles"
- Upgrading to Laravel 8.x
- Get The Count of the Number of Users in an AD Group
- Multiple Vagrant VMs in One Vagrantfile
- Fixing the "this is larger than GitHub's recommended maximum file size of 50.00 MB" error
- Changing the Directory Vagrant Stores the VMs In
- Accepting Android SDK Licenses From The OSX Command Line
- Fixing the 'Target class [config] does not exist' Error
- Using Rectangle to Manage MacOS Windows